In 1972, C was developed at Bell Laboratories by Dennis Ritchie.
- C is a simple programming language with a relatively simple to understand syntax and few keywords.
C is useless.C itself has no input/output commands, doesn't have support for strings as a fundamental data type. There is no useful math functions built in.
C requires the use of libraries as C is useless by itself. This increases the complexity of the C.The use of ANSI libraries and other methods,the issue of standard libraries is resolved.
C Programming :: A Quick Hellow World Program
Let's give a simple program that prints out "Hello World" to standard out. We'll call our program as hello.c.#include
main() {
printf("Hello, world!\n");
return 0;
}
Explanation of The Above Code:
#include-This line tells the compiler to include this header file for compilation.
What is header file?They contain prototypes and other compiler/pre-processor directive.Prototypes are also called the basic abstract function definitions.
Some common header files are stdio.h,stdlib.h, unistd.h and math.h.
- main()- This is a function, in particular it is the main block.
{ } - These curly braces are equivalent to the stating that "block begin" and "block end".These can be used at many places,such as switch and if statement.
printf() - This is the actual print statement which is used in our c program fraquently.we have header file stdio.h! But what it does? How it is defined?
- return 0-What is this? Who knows what is this
Then the return 0 statement. Seems like we are trying to give something back, and it gives the result as an integer. Maybe if we modified our main function definition: int main() ,now we are saying that our main function will be returning an integer!So,you should always explicitly declare the return type on the function.
Let us add #includeto our includes. Let's change our original return statement to return EXIT_SUCCESS;. Now it makes sense!
printf always returns an int. The main pages say that printf returns the number of characters printed.It is good programming practice to check for return values. It will not only make your program more readable, but at the end it will make your programs less error prone. But we don't really need it in this particular case.So we cast the function's return to (void). fprintf,exit and fflush are the only functions where you should do this.
What about the documentation? We should probably document some of our code so that other people can understand what we are doing. Comments in the C89 standard are noted by this: /* */. The comment always begins with /* and ends with */.
An Improved Code of The Above Example
#include #include
/* Main Function
* Purpose: Controls our program, prints Hello, World!
* Input: None
* Output: Returns Exit Status
*/
int main() {
(void)printf("Hello, world!\n");
return EXIT_SUCCESS;
}
Note:
The KEY POINT of this whole introduction is to show you the fundamental difference between understandability and correctness. If you lose understandability in an attempt to gain correctness, you will lose at the end. Always place the understandability as a priority ABOVE correctness. If a program is more understandable in the end,the chances it can be fixed correctly will be much higher. It is recommend that you should always document your program. You stand less of a chance of screwing up your program later,if you try to make your program itself more understandable.
The advantages of C
In other words,for writing anything from small programs for personal amusement to complex industrial applications,C is one of a large number of high-level languages designed for general-purpose programming.C has many advantages:
The C Compilation Model
Creating, Compiling and Running Your Program
Creating the program
Compilation
Running the program
The next stage is to run your executable program.You simply type the name of the file containing it, in this case program (or a.out),to run an executable in UNIX.This executes your program able to print any results to the screen. At this stage there may be run-time errors, such as it may become evident that the program has produced incorrect output or division by zero.If so, you must return to edit your program source, and compile it again, and run it again.
C is a High Level Language
C is also called as a high-level language. To give a list of instructions (a computer program) to a computer,the high-level computer language is used. The native language of the computer is a stream of numbers called machine level language. As you might expect, the action resulting from a single machine language instruction is very primitive, and many thousands of them can be required to do something like substantial. A high-level language provides a set of instructions you can recombine creatively and give to the imaginary black boxe of the computer. The high-level language software will then translate these high-level instructions into the low-level machine language instructions
Characteristics of C
We briefly list some of C's characteristics that have lead to its popularity as a programming language and define the language. Naturally we will be studying many of these aspects throughout our tutorial.
- Extensive use of function calls
- Small size
- Loose typing -- unlike PASCAL
- Structured language
- Low level (BitWise) programming readily available
Pointer implementation - extensive use of pointers for memory, array, structures and functions.
- It has high-level constructs.
- It produces efficient programs.
- It can handle low-level activities.
- It can be compiled on a variety of computers.
The main drawback of c is that it has poor error detection which can make it off putting to the beginner. However diligence in this matter can pay off handsomely since having learned the rules of the C we can break them. Not all languages allow this. This if done carefully and properly leads to the power of C programming.
C Program Structure
A C program basically has the following form:- Preprocessor Commands
Function prototypes -- declare function types and variables passed to function.- Type definitions
- Variables
- Functions
We must have a main() functionC assumes that function returns an integer type,if the type definition is omitted. NOTE: This can be a source of the problems in a program/* Sample program */
main()
{
printf( ``I Like C \n'' );
exit ( 0 );
}NOTE:
- printf is a standard C function -- called from main.
- C requires a semicolon at the end of the every statement.
- \n signifies newline. Formatted output -- more later.
-
exit() is also a standard function that causes the program to terminate. Strictly speaking it is not needed here as it is the last line of main() and the program will terminate anyway.
Introduction to C Programming
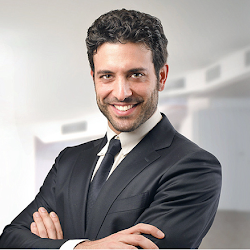
#vpsinghrajput
Author & Editor
Has laoreet percipitur ad. Vide interesset in mei, no his legimus verterem. Et nostrum imperdiet appellantur usu, mnesarchum referrentur id vim.
4:11 AM
Subscribe to:
Post Comments (Atom)
0 comments:
Post a Comment